before i twiddle with r12/r13:
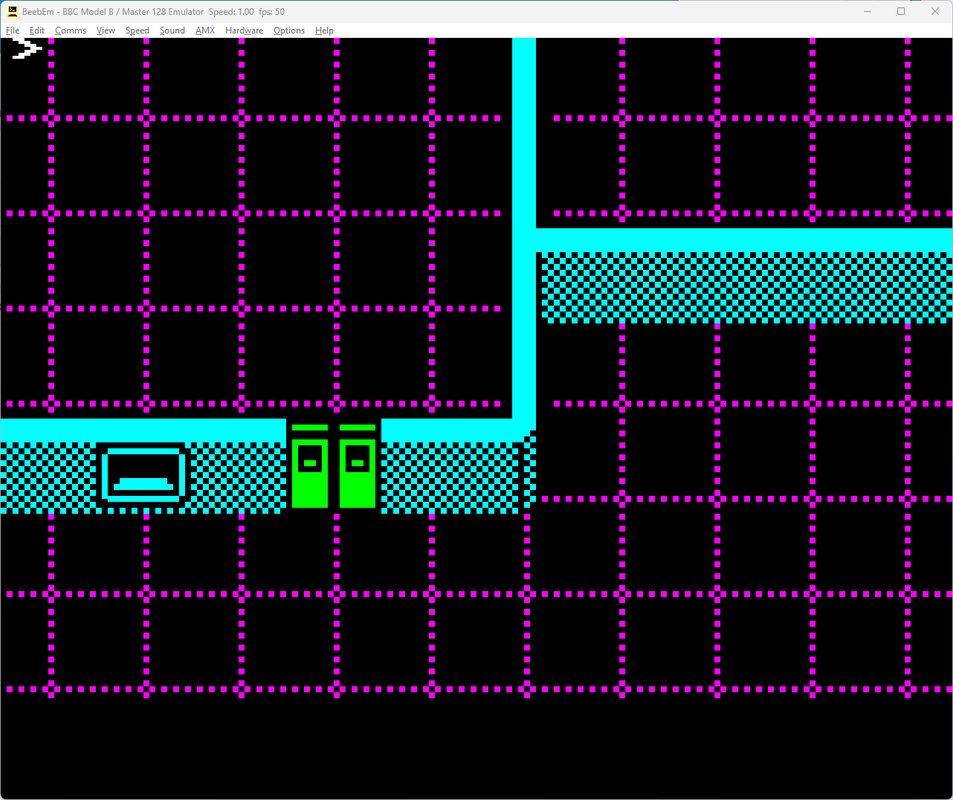
after i do so:
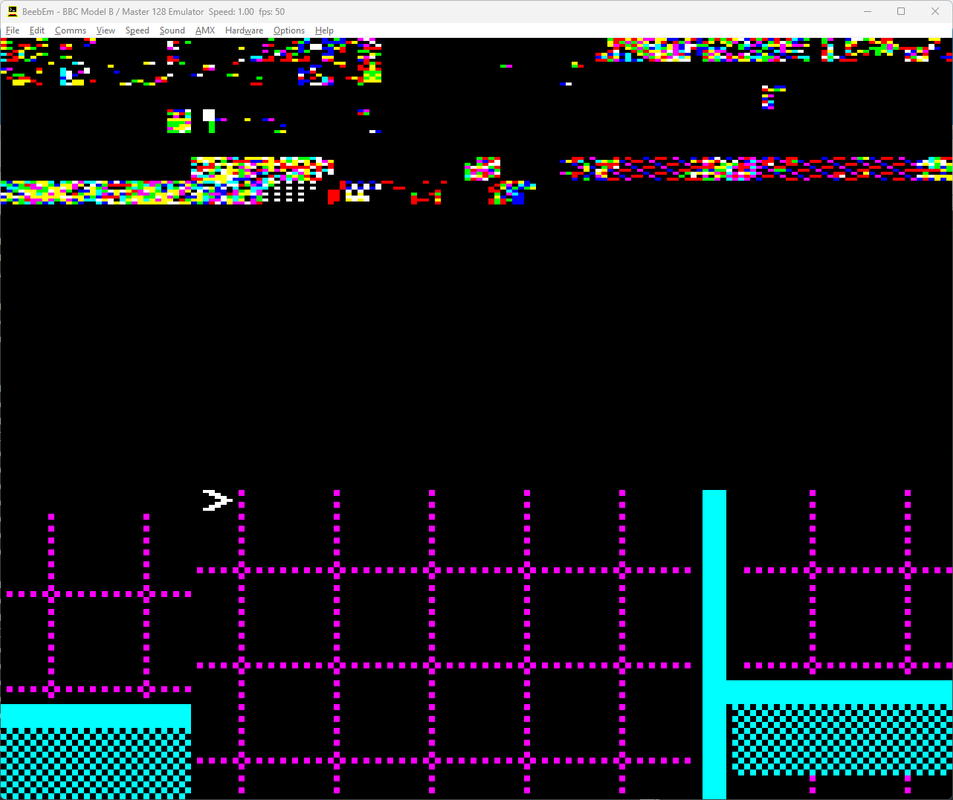
Code: Select all
; pointers to MOS
romsel = &FE30
osasci = &FFE3
oswrch = &FFEE
osbyte = &FFF4
oscli = &FFF7
romselcopy = &F4
; zero page addresses
addr = &70 ; +71
screen = &72 ; +73
counter1 = &74
counter2 = &75
counter3 = &76
counter4 = &77
xpos = &78
ypos = &79
counter5 = &80
; Code origin - PAGE is always E00 on a Master
ORG &E00
; Put a guard at the start of screen memory in mode 2
GUARD &3000
; Macros
MACRO VSYNC
LDA #19:JSR osbyte
ENDMACRO
.mainstart
; switch to mode 2 (shadow on - mode 130) (VDU 22,130)
LDA #22:JSR oswrch
;LDA #130:JSR oswrch
LDA #2:JSR oswrch
; load our SWRAM into bank 4, by calling OSCLI with "command"
LDX #LO(command)
LDY #HI(command)
JSR oscli
; page in swram bank 4
SEI:LDA #4:STA romselcopy:STA romsel:CLI
; disable cursor (VDU 23,1,0,0,0,0,0,0,0,0)
LDA #23:JSR oswrch
LDA #1:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
; disable cursor editing (*FX 4,1)
LDA #4:LDX #1:JSR osbyte
; select main memory for VDU access (*FX 112,1)
;LDA #112:LDX #1:JSR osbyte
; clear screen (VDU 16)
;LDA #16:JSR oswrch
;LDA #12:JSR oswrch
; select main memory for display (*FX 113.1)
;LDA #113:LDX #1:JSR osbyte
; access shadow memory at &3000 (*FX 108,1)
;LDA #108:LDX #1:JSR osbyte
; LDA #1
; LDX #4
; LDY #6
; JSR drawsprite
LDA #69
STA counter5
LDA #18
STA counter3
LDA #24
STA counter4
.drawtiles
.draw
LDX counter5
LDA map, X
LDX counter3
LDY counter4
JSR drawsprite
DEC counter5
DEC counter3:DEC counter3
BPL draw
LDA #18
STA counter3
DEC counter4:DEC counter4:DEC counter4:DEC counter4
BPL drawtiles
LDA #23:JSR oswrch
LDA #0:JSR oswrch
LDA #12:JSR oswrch
LDA #HI(&3280 DIV 8)
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #23:JSR oswrch
LDA #0:JSR oswrch
LDA #13:JSR oswrch
LDA #LO(&3280 DIV 8)
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
LDA #0:JSR oswrch
; LDA #0
; STA counter3
; LDA #12
; STA counter4
; .animate
; LDX counter3
; LDY counter4
; JSR drawsprite
; VSYNC
;
; LDA counter3
; CMP #18
; BEQ end
;
; LDX counter3
; LDY counter4
; JSR drawtile
;
; LDA counter3
; CLC
; ADC #2
; STA counter3
; JMP animate
; .end
; select shadow memory for display (*FX 113,2)
;LDA #113:LDX #2:JSR osbyte
RTS
.drawsprite ; pass A, X and Y
{
STA counter2
; number of sprite rows
LDA #4
STA counter1
; position of sprite
STX xpos
STY ypos
; get pointer to sprite
LDA #LO(sprite)
STA addr
LDA #HI(sprite)
STA addr+1
LDY counter2
LDA addr
CLC
ADC tileoffsetslo, Y
STA addr
LDY counter2
LDA addr+1
ADC tileoffsetshi, Y
STA addr+1
; draw 1/4 of the sprite
.plotpixels
{
LDY ypos
LDA loscreen,Y
STA screen
LDA hiscreen,Y
STA screen+1
LDX xpos
LDA screen
CLC
ADC lox, X
STA screen
LDA screen+1
ADC hix, X
STA screen+1
LDY #63
.loop
LDA (addr),Y
STA (screen),Y
DEY
BPL loop
}
; increase the sprite pointer
LDA addr
CLC
ADC #64
STA addr
LDA addr+1
ADC #0
STA addr+1
; increase the screen row
INC ypos
; loop through rows
DEC counter1
BNE plotpixels
}
RTS
.drawtile ; pass X and Y registers
{
; get pointer to sprite
LDA #LO(sprite)
STA addr
LDA #HI(sprite)
STA addr+1
; number of sprite rows
LDA #4
STA counter1
; position of sprite
STX xpos
STY ypos
; draw 1/4 of the sprite
.plotpixels
{
LDY ypos
LDA loscreen,Y
STA screen
LDA hiscreen,Y
STA screen+1
LDX xpos
LDA screen
CLC
ADC lox, X
STA screen
LDA screen+1
ADC hix, X
STA screen+1
LDY #63
.loop
LDA (addr),Y
STA (screen),Y
DEY
BPL loop
}
; increase the sprite pointer
LDA addr
CLC
ADC #64
STA addr
LDA addr+1
ADC #0
STA addr+1
; increase the screen row
INC ypos
; loop through rows
DEC counter1
BNE plotpixels
}
RTS
.hiscreen EQUB &30, &32, &35, &37, &3A, &3C, &3F, &41, &44, &46, &49, &4B, &4E, &50, &53, &55, &58, &5A, &5D, &5F, &62, &64, &67, &69, &6C, &6E, &71, &73, &76, &78, &7B, &7D
.loscreen EQUB &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80, &00, &80
.hix EQUB &00, &00, &00, &00, &00, &00, &00, &00, &01, &01, &01, &01, &01, &01, &01, &01, &02, &02, &02, &02
.lox EQUB &00, &20, &40, &60, &80, &A0, &C0, &E0, &00, &20, &40, &60, &80, &A0, &C0, &E0, &00, &20, &40, &60
.map EQUB &00, &00, &00, &00, &00, &03, &00, &00, &00, &00
EQUB &00, &00, &00, &00, &00, &03, &00, &00, &00, &00
EQUB &00, &00, &00, &00, &00, &04, &01, &01, &01, &01
EQUB &00, &00, &00, &00, &00, &03, &00, &00, &00, &00
EQUB &01, &06, &01, &05, &01, &02, &00, &00, &00, &00
EQUB &00, &00, &00, &00, &00, &00, &00, &00, &00, &00
EQUB &00, &00, &00, &00, &00, &00, &00, &00, &00, &00
.tileoffsetslo EQUB &00, &00, &00, &00, &00, &00, &00
.tileoffsetshi EQUB &00, &01, &02, &03, &04, &05, &06
.command EQUS "SRLOAD SWRAM 8000 4", 13
.mainend
SAVE "Code", mainstart, mainend
; ********************
; SWRAM
; ********************
; start of sideways bank
ORG &8000
; guard end of bank
GUARD &C000
.swramstart
.sprite INCBIN "C:\Users\c0bur\Desktop\chips\sprites.bin"
.swramend
SAVE "SWRAM", swramstart, swramend