In this thread I'll be working on converting this to BBC Basic as part of my relearning of this language.
The aim of the game is this:-
For input, the player puts in an initial X position, velocity and starting angle to try and set the Space Probe off towards the exit goal, which will be randomly placed on the far side of the screen (a simple line). If the space probe is plotted into the exit goal area then you've won the game. The graduated axis on the left is to aid the player on deciding that starting position - though I've yet to add some number against the divisions to help with that. The planet will also be placed randomly on the screen with a random size which in turn will affect it's mass. The game demonstrates the interaction of the gravitational force between two bodies.
For anyone else that may have published their BASIC programs during that time, there's two useful archives here:-
1. Index for Spectrum/QL typed in scripts : http://www.users.globalnet.co.uk/~jg27p ... _names.htm
2. This archive is from the "World of Spectrum" but the mag covers all scripts from different machines of the time. https://worldofspectrum.org/archive/mag ... ing-weekly
Here's the original mag and published page:-
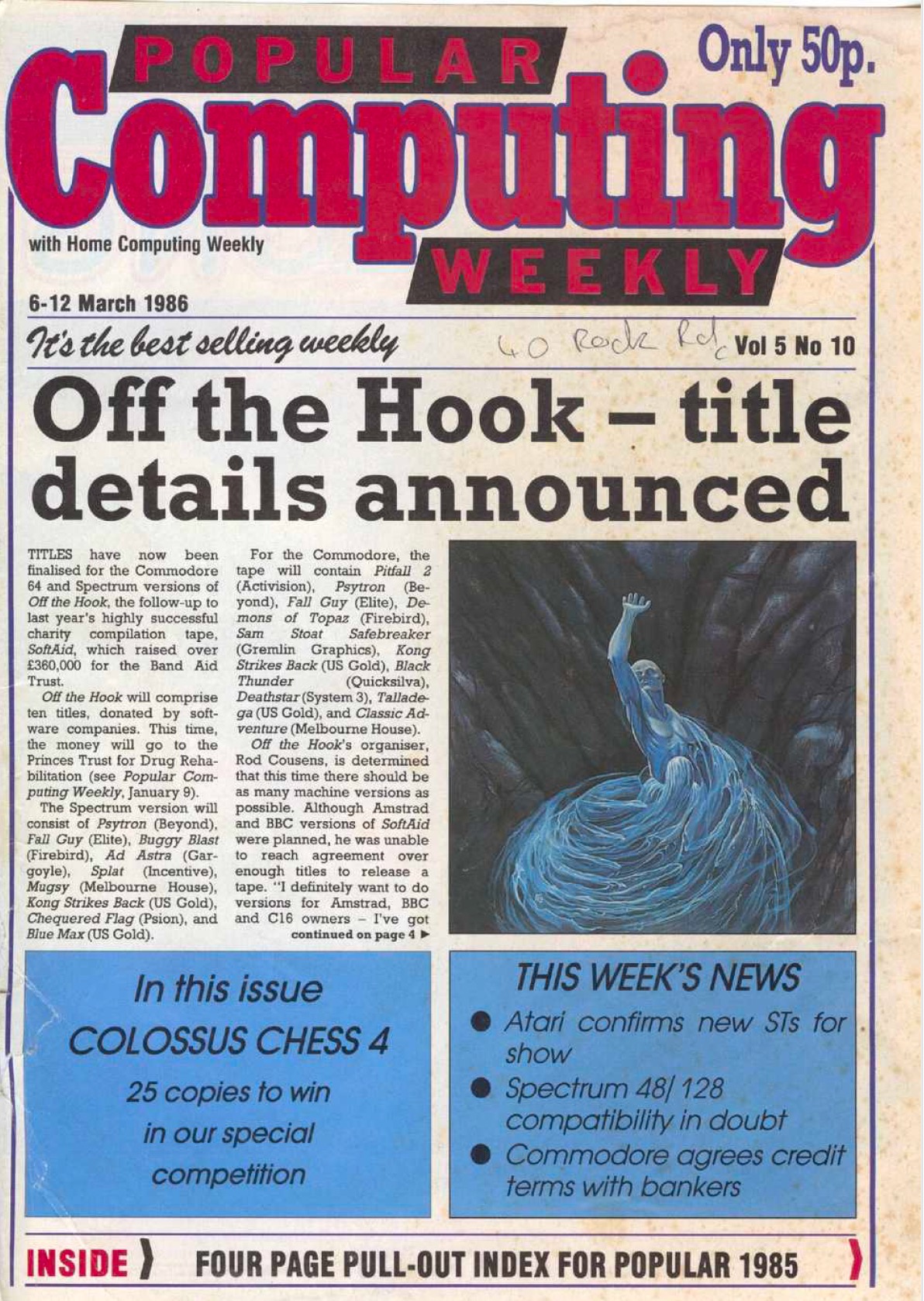

I've been using the excellent Owlet editor on my Mac (I can type faster on a modern keyboard) and my first question is on the use of RND, which is very much prevalent in the code above which I'll need in the BBC script.
I've noticed in Owlet that when using RND(X) it ends up giving you the same number each time. On my actual BBC B it does give random numbers each time (pseudo random anyway). So how to test the code in Owlet? Is there something I can do about this or is there another editor I can use that doesn't have this limitation.
Here's my script so far (still needs work) which doesn't use RND at the moment because of the restriction above.
Code: Select all
MODE 1
CLS
PROC_Initialise
:REM Drawing Saturn (planet body) as a circle in the center
saturnX% = 640
saturnY% = 512
saturnRadius% = 240
PROC_DrawPlanet(saturnX%, saturnY%, saturnRadius%)
:REM Drawing Saturn's Rings using filled ellipses
GCOL 0, 3
FOR ringNum% = 1 TO 3
ringWidth% = saturnRadius% + ringNum% * 70
ringHeight% = 20 + ringNum% * 12
PROC_DrawEllipse(saturnX%, saturnY%, ringWidth%, ringHeight%)
NEXT
:REM Initialize spacecraft's position and velocity for slingshot trajectory
spacecraftX% = 200
spacecraftY% = 750
vx% = 2
vy% = -3
:REM Simulate and draw spacecraft's path
GCOL 0, 7 :REM Color for spacecraft
FOR t% = 1 TO 2000
PROC_DrawSpacecraft(spacecraftX%, spacecraftY%)
:REM Compute gravitational force
dx% = saturnX% - spacecraftX%
dy% = saturnY% - spacecraftY%
distance% = SQR(dx%^2 + dy%^2)
:REM For simplicity, we'll use a scaled gravitational constant
G% = 1E-3
force% = G% / distance%^2
:REM Adjust velocity based on gravitational force
accelerationX% = (force% * dx% / distance%)
accelerationY% = (force% * dy% / distance%)
vx% = vx% + accelerationX%
vy% = vy% + accelerationY%
:REM Update spacecraft's position
spacecraftX% = spacecraftX% + vx%
spacecraftY% = spacecraftY% + vy%
:REM IF distance% < saturnRadius% THEN EXIT
NEXT
STOP
DEF PROC_Initialise
VDU 23,1,0;0;0;0;
COLOUR 0
COLOUR 1
ENDPROC
DEF PROC_DrawPlanet(h%, k%, r%)
GCOL 0, 5
MOVE h%, k%
PLOT 145, r%, 0
ENDPROC
DEF PROC_DrawEllipse(h%, k%, hr%, vr%)
MOVE h%, k%
PLOT 0, hr%, 0
PLOT 193, 0, vr%
ENDPROC
DEF PROC_DrawSpacecraft(x%, y%)
PLOT 69, x%, y%
ENDPROC